Kids Spelling VR
A game for kids to arrange the order of unordered letter boxes, the order should form a noun and if its correct, he gains score.
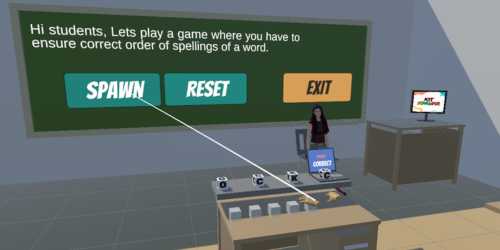.png)
CODE
public class GameManager : MonoBehaviour
{
private string word;
public Transform Socket_Position, Boxes_Position;
private Vector3 oldPosition_Box, oldPosition_Socket;
private List<string> list1, list2;
public string spell_word;
List<string> nouns = new List<string>
{
"cat", "dog", "bird", "fish", "tree", "rock", "star", "coin",
"book", "car", "pen", "mouse", "phone", "house", "chair", "key",
"shoe", "sock", "ball", "lamp", "cup", "note", "ring", "fork",
"spoon", "brush", "plate" , "clock", "table", "dress", "pencil",
"paper", "plant", "shell", "map", "hat", "stick", "path", "cloud"
};
public List<GameObject> Boxes = new List<GameObject>();
public List<GameObject> Sockets_Placer = new List<GameObject>();
bool press = true;
void Start()
{
oldPosition_Box = Boxes_Position.position;
oldPosition_Socket = Socket_Position.position;
}
public string GetRandomNoun()
{
System.Random random = new System.Random();
int index = random.Next(nouns.Count);
spell_word = nouns[index];
return spell_word;
}
void Update()
{
}
public static string ShuffleString(string s)
{
System.Random rand = new System.Random();
return new string(s.ToCharArray().OrderBy(c => rand.Next()).ToArray());
}
public void Spawn_Button()
{
if (press == true)
{
string randomword = GetRandomNoun();
string shuffleword = ShuffleString(randomword);
Generate_Word(shuffleword);
Generate_Socket(randomword);
press = false;
}
}
public void Generate_Word(string word)
{
Boxes_Position.position = oldPosition_Box;
Vector3 offset = new Vector3(0.4f, 0, 0);
for (int i = 0; i < word.Length; i++) {
char temp = word[i];
int index = temp - 'a';
Instantiate(Boxes[index], Boxes_Position.position, Boxes_Position.rotation);
Boxes_Position.position -= offset;
}
}
public void Generate_Socket(string word)
{
Socket_Position.position = oldPosition_Socket;
Vector3 offset = new Vector3(0.2f, 0, 0);
for (int i = 0; i < word.Length; i++)
{
char temp = word[i];
int index = temp - 'a';
Instantiate(Sockets_Placer[index], Socket_Position.position, Socket_Position.rotation);
Socket_Position.position -= offset;
}
}
public void check_Spelling()
{
Socket sockett = new Socket();
List<char> submited = sockett.Word();
Debug.Log(submited);
}
public string Spell_word()
{
return spell_word;
}
public void Exit()
{
Application.Quit();
}
public void ResetScene()
{
Scene currentScene = SceneManager.GetActiveScene();
SceneManager.LoadScene(currentScene.name);
}